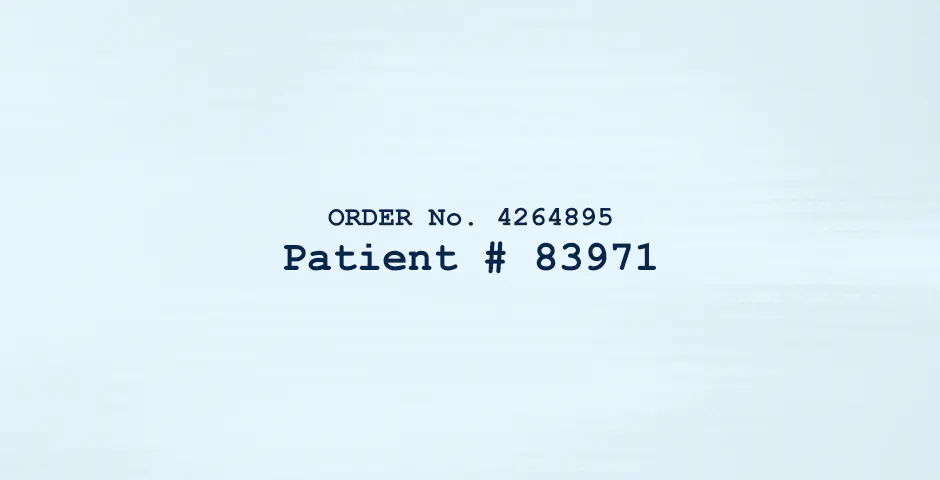
Generate unique random ID in PHP MySQL
In this short guide, I am going to show you how to generate a unique random ID in PHP MySQL. What is a unique ID? There will be a time when you or your client would need to have a random and especially unique ID (identification) in a web application.
For example, for an ecommerce site, there will be order IDs assigned to each order. For a school, there will be student IDs assigned to each student, and the same for a health center or hospital. A hospital would also need unique IDs assigned to their patients. The important thing which matters here is the uniqueness because the same one ID cannot be assigned to multiple records.
e.g. Order No. 897869 Patient # 845271 or student ID 3849
So, in this tutorial, we will accomplish two things. First, we will generate a random ID, and then we will make sure this ID is unique. Let’s begin and see how to generate unique random ID in PHP MySQL
How to generate a random ID in PHP
It’s up to you to set the format of the ID. From setting the length to making it all numbers, all alphabets, or mix. We will cover both, first we will generate numbers only and then mix.
function generate_random_number($min = 0, $max = 9) {
return rand($min, $max);
}
echo generate_random_number();
This above function will generate any random number between 0 and 9. If we change the max value to 1000, it will then generate any random number between 0 and 1000.
In case, we want to fix the length of the number, then we will set the min value like the following example. Let’s generate an eight digits number.
function generate_random_number($min = 10000000, $max = 99999999) {
return rand($min, $max);
}
echo generate_random_number();
Now it will generate a nice eight digit random number for us. You can read more about PHP’s rand() function here. Let’s see, quickly, how we can generate numbers and letters mixed ID.
function generate_random_mix($length = 8) {
$letters = "abcdefghijklmnopqrstuvwxyz0123456789";
return substr(str_shuffle($letters), 0, $length);
}
echo generate_random_mix();
Now we will have a random but mixed characters ID, for example 3m0e84px. BTW, YouTube also uses a mixed ID for their videos. Now let’s see how to make it unique.
How to make Unique ID in PHP
function generate_unique_id(){
// your database connection variable.
global $con;
// Generate a randum number
$id = generate_random_number();
// Check if that number alrady exists in the databse.
$check = mysqli_query($con, "SELECT 1 FROM `table_name` WHERE `id` = '".$code."' ");
// Keep regenerating and checking until the id is unique.
if (mysqli_num_rows($rand_check) == 1) {
do {
$id = generate_random_number();
} while(mysqli_num_rows($check) != 1);
}
return $id;
}
echo generate_unique_id();
Using the above code, you will have a unique ID in your website or database.
The $con variable in the function is coming from your database connection. You can learn more about how to connect to MySQL database in PHP here. That’s it for today, if you have any questions, please feel free to let me know in the comments below.