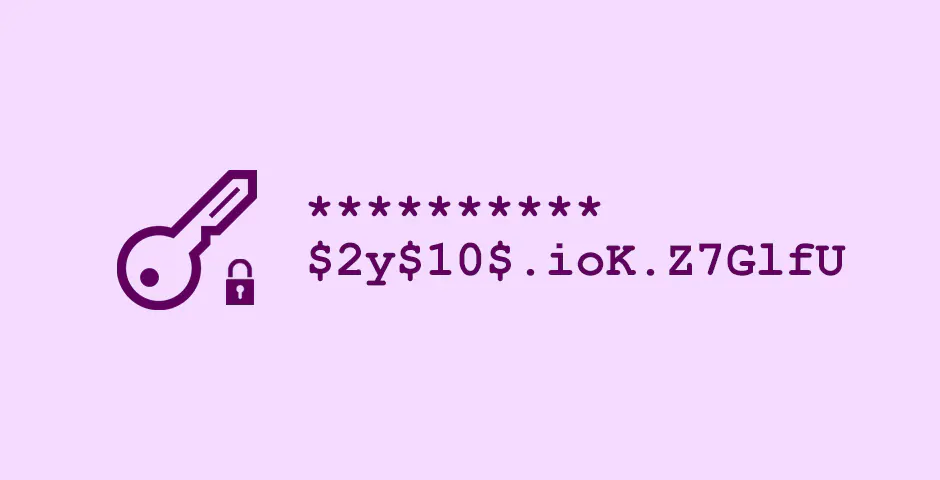
Prepare passwords to store in database in PHP
For all the websites which offer any kind of registration on their websites, they have to store user’s information into their database. So, in this short guide, I am going to show you how to prepare passwords to store in database in PHP using the recommended, efficient, and a secure method.
The main concern is the security, because we simply cannot store user’s sensitive information in a plain text. So what do we do then? The answer is, we only store the hash of the password. In simple words, we convert or encrypt the password into a hash first and then store that hashed / encrypted password into the database.
Plain Password (What user inputs)
myhero321
Hashed Password (What is stored)
$2y$10$.ioK.Z7GlfUroNbnBrDK8.5Wj03MAmHAohny9WWLnB9Igtaj64Wpq
That way the stored password is secure, so in case of any data breach, the hackers can only get to the hashed version of the password, but they cannot decrypt or read the password. Even you or the owner of the website who has all the access cannot read such passwords.
In case you are wondering, the hacker can decrypt the password but only if the password is encrypted using the absolute or poor encryption methods such as md5 or sha1. Now, let’s see, how we can prepare passwords to store in database in PHP.
How to Hash password in PHP
Thanks to the ongoing development and upgrades in PHP, we now have a special PHP function “password_hash” which makes it easy for us and do the job perfectly.
// Simple password you received from the user.
$password = "myhero321";
// Hashed password which you will store into database.
$hashed_password = password_hash($password, PASSWORD_DEFAULT);
How to read password Hash in PHP
Now we also need to read the hashed password. Technically, we are not going to read it but only going to verify it again using a PHP’s function “password_verify”.
$verify = password_verify($password, $hashed_password);
if ($verify){
// Password is correct
} else {
// Incorrect password
}
Variable $password is what user has put in on the login page and $hashed_password is what is stored on your database. Take those and verify using the method mentioned above.
That’s it. I would recommend everyone to use the best and secure methods when dealing with user’s sensitive information. Not only it’s secure, but also morally correct. You can read more about these PHP functions password_hash and password_verify.
Thank you for reading. I will keep this page updated in case there are further method introduced in the near future. In the meantime, let’s take a look at how to connect to MySQL database in PHP.