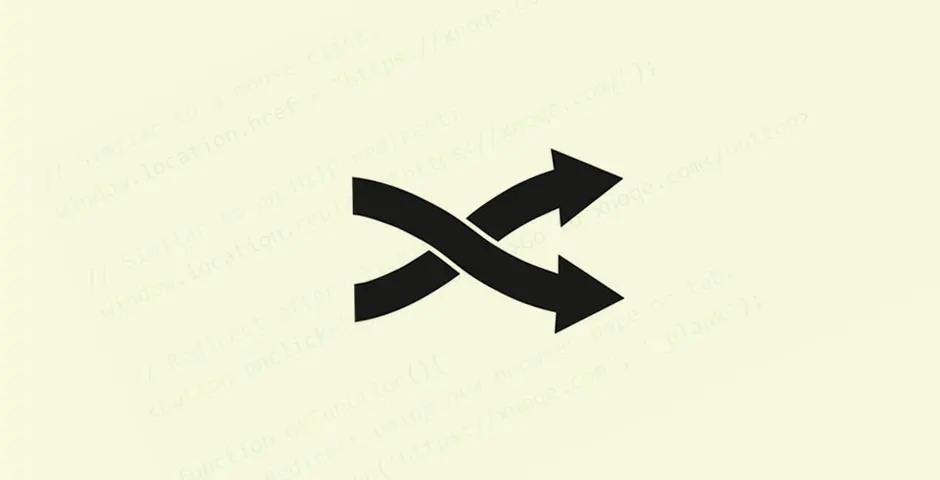
Manage user redirect on the website (HTML, PHP, JavaScript, and .HTACCESS)
Welcome to this tutorial. Today, we are going to see how can we manage user redirect on the website using HTML, PHP, JavaScript, and .HTACCESS. There will be several times or reasons where you would need to redirect a user to another page in your website or any web application.
For example, the most common is, a user may need to be redirected to a specific page right after they log in, the website or webpage is moved to a new domain or location, or if a page is temporarily unavailable, then the website should redirect a user / visitor to another page.
Here is a quick overview of four different methods which we are going to use to manage user redirect on the website.
How to redirect in HTML
First, let’s see how to redirect in HTML which is the most common especially for static websites. We are simply going to use the following meta tag for that.
Place the following code between the <head> and </head> tags of your HTML code.
<meta http-equiv="refresh" content="0; url=https://xnoqe.com/"></code>
The zero “0” mentioned in the content attribute represents the delay (in seconds) before initiating a redirect, and “url=” is the destination location where we will redirect the user to i.e. xnoqe.com
The following meta tag will redirect the user, but after waiting 5 seconds.
<meta http-equiv="refresh" content="5; url=https://xnoqe.com/"></code>
How to redirect in JavaScript
Now let’s see the same redirection method but using JavaScript instead.
// Similar to a mouse click:
window.location.href = "https://xnoqe.com/";
// Similar to an HTTP redirect:
window.location.replace("https://xnoqe.com/");
// Redirect after clicking on a button
<button onclick="myFunction()">Go to xnoqe.com</button>
function myFunction(){
// Redirect using new browser page or tab.
window.open('https://xnoqe.com', '_blank');
}
You can read more about this redirect method in JavaScript on w3schools website.
How to redirect in PHP
We use header function in PHP to manage this redirect.
header("Location: https://xnoqe.com");
To make our code efficient and reusable, we can create our own function for the redirect.
function redirect($url = 'https://xnoqe.com'){
header("Location: ".$url);
exit;
}
// This will redirect to xnoqe.com
redirect();
// This will redirect to google.com
redirect('https://www.google.com');
Using this method can sometimes give an error (headers already sent) if this method is used later down the script when any HTML code is already loaded. So, to make this even more efficient, we can combine PHP’s header and HTML’s meta tag to counter this error.
function redirect($url, $delay=0){
if (!headers_sent()){
header('Location: '.$to);
} else {
echo "<meta http-equiv='refresh' content='".$delay."; url=".$url."'>";
}
exit;
}
You can read more about headers_sent() here.
In case you have noticed that we used exit at the end of the function because we don’t want the script to load any further after a redirect is initiated. First, there is no point to load the script any further and secondly, this is better for website performance as well.
How to redirect using .htaccess
Now at the last, let’s quickly see how we can redirect using .htaccess file. We are going to use a simple line of code. That code needs to be placed inside .htaccess file. .htaccess file is located on the root level of your website.
RewriteEngine On
Redirect https://xnoqe.com/
There are many different ways we can manage user redirect on the website in .htaccess, which we will cover in a detailed separate article for .htaccess. Now let’s see, we to initiate a redirect when loading a specific page.
RewriteEngine On
Redirect /oldpage/ https://xnoqe.com/
RewriteEngine On
Redirect /oldpage/ /newpage/
That’s it for this tutorial. If you want to learn more about htaccess, I will create more detailed articles for that, so don’t forget to check the specific htaccess tag on the website and stay tuned for more upcoming tutorials. If you still have any questions, please feel free to let me know in the comments.